그냥 게임개발자
pair와 tuple 본문
pair와 tuple은 자료구조가 아니지만 C++에서 제공하는 utility라는 라이브러리의 템플릿 클래스이다.
또한 자주사용 되기에 복습해보았다.
pair
pair는 first와 second라는 멤버변수를 가지는 클래스이며 두 가지 값을 담아야 할 때 쓴다.
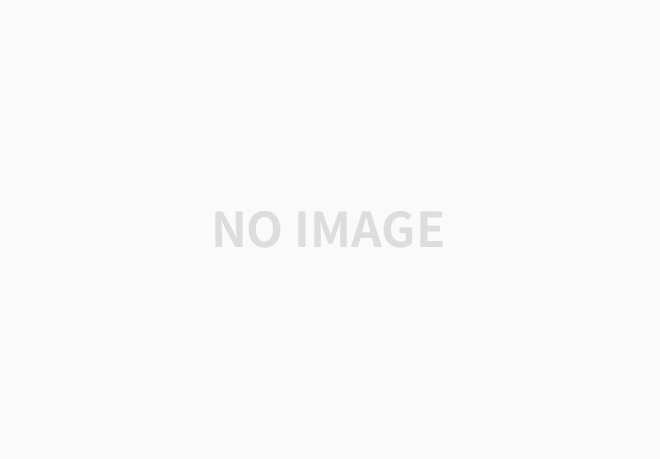
tuple
tuple은 세가지 이상의 값을 담을 때 쓰는 클래스이다.
#include <iostream>
#include <utility>
#include <tuple>
using namespace std;
pair<int, int> pi;
tuple<int, int, int> tl;
int a, b, c;
int main()
{
pi = {1, 2};
tl = make_tuple(1, 2, 3);
tie(a, b) = pi;
cout << a << " : " << b << '\n';
tie(a, b, c) = tl;
cout << a << " : " << b << " : " << c << '\n';
return 0;
}
tie를 써서 pair이나 tuple로부터 값을 끄집어내는 코드
pair의 경우 {a, b} 또는 make_pair(a, b)로도 만들 수 있다.
그저 2개의 원소를 담은 바구니를 생각하면 된다.
이 때 원래는
a = pi.first;
b = pi.second 이런식으로 끄집어내야 하는데 tie를 쓰게 되면 tie(a, b) = pi 이렇게 끄집어 낼 수가 있다.
first와 second라는 코드를 쓰지 않으니 너무나도 편하게 요소를 끄집어 낼 수 있다.
물론 이 때 a와 b는 앞서 변수로 선언되어야 한다.
pair<int, int> a = {1, 2};
// a.first = 1
// a.second = 2
int c, d;
tie(c, d) = a; // a.first, a.second를 쓰지 않고 꺼낼수가 있다.
다음은 tie를 쓰지 않고 사용하는 방식이다.
pair는 first, second로 값을 끄집어내지만
tuple의 경우 get<0>, get<int>이런식으로 값을 꺼내야 한다.
#include <iostream>
#include <utility>
#include <tuple>
using namespace std;
pair<int, int> pi;
tuple<int, int, int> ti;
int a, b, c;
int main()
{
pi = {1, 2};
a = pi.first;
b = pi.second;
cout << a << " : " << b << '\n';
ti = make_tuple(1, 2, 3);
a = get<0>(ti);
b = get<1>(ti);
c = get<2>(ti);
cout << a << " : " << b << " : " << c << '\n';
return 0;
}
결과물을 보자.
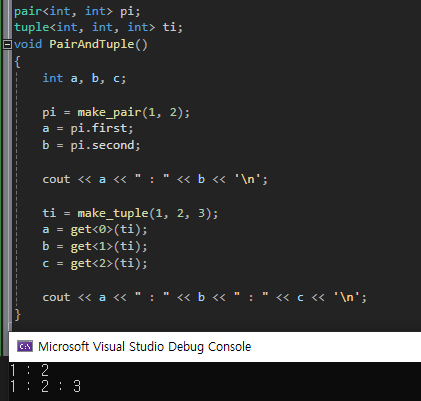
잘 결과물이 나온다.
'C++ 나만의 복습' 카테고리의 다른 글
포인터 (1) | 2024.04.05 |
---|---|
auto 타입 (0) | 2024.04.04 |
float, double (0) | 2024.04.04 |
long long (0) | 2024.04.04 |
오버플로, 언더플로 (0) | 2024.04.04 |